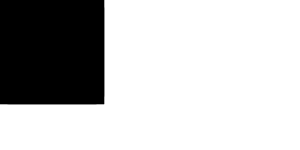
Previously, we included a redundant prefix on the panic message and a postfix of the location of the panic. The prefix didn't carry any additional information beyond "something failed", and the location of the panic is redundant with the diagnostic's span, which gets printed out even if its code is not shown. ``` error[E0080]: evaluation of constant value failed --> $DIR/assert-type-intrinsics.rs:11:9 | LL | MaybeUninit::<!>::uninit().assume_init(); | ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ evaluation panicked: aborted execution: attempted to instantiate uninhabited type `!` ``` ``` error[E0080]: evaluation of `Fail::<i32>::C` failed --> $DIR/collect-in-dead-closure.rs:9:19 | LL | const C: () = panic!(); | ^^^^^^^^ evaluation panicked: explicit panic | = note: this error originates in the macro `$crate::panic::panic_2015` which comes from the expansion of the macro `panic` (in Nightly builds, run with -Z macro-backtrace for more info) ``` ``` error[E0080]: evaluation of constant value failed --> $DIR/uninhabited.rs:41:9 | LL | assert!(false); | ^^^^^^^^^^^^^^ evaluation panicked: assertion failed: false | = note: this error originates in the macro `assert` (in Nightly builds, run with -Z macro-backtrace for more info) ``` --- When the primary span for a const error is the same as the first frame in the const error report, skip it. ``` error[E0080]: evaluation of constant value failed --> $DIR/issue-88434-removal-index-should-be-less.rs:3:24 | LL | const _CONST: &[u8] = &f(&[], |_| {}); | ^^^^^^^^^^^^^^ evaluation panicked: explicit panic | note: inside `f::<{closure@$DIR/issue-88434-removal-index-should-be-less.rs:3:31: 3:34}>` --> $DIR/issue-88434-removal-index-should-be-less.rs:10:5 | LL | panic!() | ^^^^^^^^ the failure occurred here = note: this error originates in the macro `$crate::panic::panic_2015` which comes from the expansion of the macro `panic` (in Nightly builds, run with -Z macro-backtrace for more info) ``` instead of ``` error[E0080]: evaluation of constant value failed --> $DIR/issue-88434-removal-index-should-be-less.rs:10:5 | LL | panic!() | ^^^^^^^^ explicit panic | note: inside `f::<{closure@$DIR/issue-88434-removal-index-should-be-less.rs:3:31: 3:34}>` --> $DIR/issue-88434-removal-index-should-be-less.rs:10:5 | LL | panic!() | ^^^^^^^^ note: inside `_CONST` --> $DIR/issue-88434-removal-index-should-be-less.rs:3:24 | LL | const _CONST: &[u8] = &f(&[], |_| {}); | ^^^^^^^^^^^^^^ = note: this error originates in the macro `$crate::panic::panic_2015` which comes from the expansion of the macro `panic` (in Nightly builds, run with -Z macro-backtrace for more info) ``` --- Revert order of constant evaluation errors Point at the code the user wrote first and std functions last. ``` error[E0080]: evaluation of constant value failed --> $DIR/const-errs-dont-conflict-103369.rs:5:25 | LL | impl ConstGenericTrait<{my_fn(1)}> for () {} | ^^^^^^^^ evaluation panicked: Some error occurred | note: called from `my_fn` --> $DIR/const-errs-dont-conflict-103369.rs:10:5 | LL | panic!("Some error occurred"); | ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ = note: this error originates in the macro `$crate::panic::panic_2015` which comes from the expansion of the macro `panic` (in Nightly builds, run with -Z macro-backtrace for more info) ``` instead of ``` error[E0080]: evaluation of constant value failed --> $DIR/const-errs-dont-conflict-103369.rs:10:5 | LL | panic!("Some error occurred"); | ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ Some error occurred | note: called from `<() as ConstGenericTrait<{my_fn(1)}>>::{constant#0}` --> $DIR/const-errs-dont-conflict-103369.rs:5:25 | LL | impl ConstGenericTrait<{my_fn(1)}> for () {} | ^^^^^^^^ = note: this error originates in the macro `$crate::panic::panic_2015` which comes from the expansion of the macro `panic` (in Nightly builds, run with -Z macro-backtrace for more info) ```
70 lines
2.7 KiB
Rust
70 lines
2.7 KiB
Rust
// ignore-tidy-linelength
|
|
// Strip out raw byte dumps to make comparison platform-independent:
|
|
//@ normalize-stderr: "(the raw bytes of the constant) \(size: [0-9]*, align: [0-9]*\)" -> "$1 (size: $$SIZE, align: $$ALIGN)"
|
|
//@ normalize-stderr: "([0-9a-f][0-9a-f] |╾─*ALLOC[0-9]+(\+[a-z0-9]+)?(<imm>)?─*╼ )+ *│.*" -> "HEX_DUMP"
|
|
#![allow(invalid_value)]
|
|
|
|
use std::mem;
|
|
|
|
#[repr(C)]
|
|
union MaybeUninit<T: Copy> {
|
|
uninit: (),
|
|
init: T,
|
|
}
|
|
|
|
const UNALIGNED: &u16 = unsafe { mem::transmute(&[0u8; 4]) };
|
|
//~^ ERROR it is undefined behavior to use this value
|
|
//~| constructing invalid value: encountered an unaligned reference (required 2 byte alignment but found 1)
|
|
|
|
const UNALIGNED_BOX: Box<u16> = unsafe { mem::transmute(&[0u8; 4]) };
|
|
//~^ ERROR it is undefined behavior to use this value
|
|
//~| constructing invalid value: encountered an unaligned box (required 2 byte alignment but found 1)
|
|
|
|
const NULL: &u16 = unsafe { mem::transmute(0usize) };
|
|
//~^ ERROR it is undefined behavior to use this value
|
|
|
|
const NULL_BOX: Box<u16> = unsafe { mem::transmute(0usize) };
|
|
//~^ ERROR it is undefined behavior to use this value
|
|
|
|
|
|
// It is very important that we reject this: We do promote `&(4 * REF_AS_USIZE)`,
|
|
// but that would fail to compile; so we ended up breaking user code that would
|
|
// have worked fine had we not promoted.
|
|
const REF_AS_USIZE: usize = unsafe { mem::transmute(&0) };
|
|
//~^ ERROR evaluation of constant value failed
|
|
|
|
const REF_AS_USIZE_SLICE: &[usize] = &[unsafe { mem::transmute(&0) }];
|
|
//~^ ERROR evaluation of constant value failed
|
|
|
|
const REF_AS_USIZE_BOX_SLICE: Box<[usize]> = unsafe { mem::transmute::<&[usize], _>(&[mem::transmute(&0)]) };
|
|
//~^ ERROR evaluation of constant value failed
|
|
|
|
const USIZE_AS_REF: &'static u8 = unsafe { mem::transmute(1337usize) };
|
|
//~^ ERROR it is undefined behavior to use this value
|
|
|
|
const USIZE_AS_BOX: Box<u8> = unsafe { mem::transmute(1337usize) };
|
|
//~^ ERROR it is undefined behavior to use this value
|
|
|
|
const UNINIT_PTR: *const i32 = unsafe { MaybeUninit { uninit: () }.init };
|
|
//~^ ERROR evaluation of constant value failed
|
|
//~| uninitialized
|
|
|
|
const NULL_FN_PTR: fn() = unsafe { mem::transmute(0usize) };
|
|
//~^ ERROR it is undefined behavior to use this value
|
|
const UNINIT_FN_PTR: fn() = unsafe { MaybeUninit { uninit: () }.init };
|
|
//~^ ERROR evaluation of constant value failed
|
|
//~| uninitialized
|
|
const DANGLING_FN_PTR: fn() = unsafe { mem::transmute(13usize) };
|
|
//~^ ERROR it is undefined behavior to use this value
|
|
const DATA_FN_PTR: fn() = unsafe { mem::transmute(&13) };
|
|
//~^ ERROR it is undefined behavior to use this value
|
|
|
|
|
|
const UNALIGNED_READ: () = unsafe {
|
|
let x = &[0u8; 4];
|
|
let ptr = x.as_ptr().cast::<u32>();
|
|
ptr.read(); //~ ERROR evaluation of constant value failed
|
|
};
|
|
|
|
|
|
fn main() {}
|