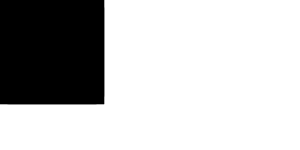
As a rule, the application of `unsafe` to a declaration requires that use-sites of that declaration also require `unsafe`. For example, a field declared `unsafe` may only be read in the lexical context of an `unsafe` block. For nearly all safe traits, the safety obligations of fields are explicitly discharged when they are mentioned in method definitions. For example, idiomatically implementing `Clone` (a safe trait) for a type with unsafe fields will require `unsafe` to clone those fields. Prior to this commit, `Copy` violated this rule. The trait is marked safe, and although it has no explicit methods, its implementation permits reads of `Self`. This commit resolves this by making `Copy` conditionally safe to implement. It remains safe to implement for ADTs without unsafe fields, but unsafe to implement for ADTs with unsafe fields. Tracking: #132922
41 lines
757 B
Rust
41 lines
757 B
Rust
//@ compile-flags: --crate-type=lib
|
|
|
|
#![feature(unsafe_fields)]
|
|
#![allow(incomplete_features)]
|
|
#![deny(missing_copy_implementations)]
|
|
|
|
mod good_safe_impl {
|
|
enum SafeEnum {
|
|
Safe(u8),
|
|
}
|
|
|
|
impl Copy for SafeEnum {}
|
|
}
|
|
|
|
mod bad_safe_impl {
|
|
enum UnsafeEnum {
|
|
Safe(u8),
|
|
Unsafe { unsafe field: u8 },
|
|
}
|
|
|
|
impl Copy for UnsafeEnum {}
|
|
//~^ ERROR the trait `Copy` requires an `unsafe impl` declaration
|
|
}
|
|
|
|
mod good_unsafe_impl {
|
|
enum UnsafeEnum {
|
|
Safe(u8),
|
|
Unsafe { unsafe field: u8 },
|
|
}
|
|
|
|
unsafe impl Copy for UnsafeEnum {}
|
|
}
|
|
|
|
mod bad_unsafe_impl {
|
|
enum SafeEnum {
|
|
Safe(u8),
|
|
}
|
|
|
|
unsafe impl Copy for SafeEnum {}
|
|
//~^ ERROR implementing the trait `Copy` is not unsafe
|
|
}
|