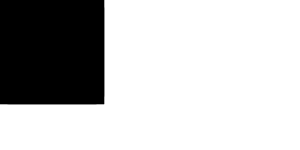
Previously, we included a redundant prefix on the panic message and a postfix of the location of the panic. The prefix didn't carry any additional information beyond "something failed", and the location of the panic is redundant with the diagnostic's span, which gets printed out even if its code is not shown. ``` error[E0080]: evaluation of constant value failed --> $DIR/assert-type-intrinsics.rs:11:9 | LL | MaybeUninit::<!>::uninit().assume_init(); | ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ evaluation panicked: aborted execution: attempted to instantiate uninhabited type `!` ``` ``` error[E0080]: evaluation of `Fail::<i32>::C` failed --> $DIR/collect-in-dead-closure.rs:9:19 | LL | const C: () = panic!(); | ^^^^^^^^ evaluation panicked: explicit panic | = note: this error originates in the macro `$crate::panic::panic_2015` which comes from the expansion of the macro `panic` (in Nightly builds, run with -Z macro-backtrace for more info) ``` ``` error[E0080]: evaluation of constant value failed --> $DIR/uninhabited.rs:41:9 | LL | assert!(false); | ^^^^^^^^^^^^^^ evaluation panicked: assertion failed: false | = note: this error originates in the macro `assert` (in Nightly builds, run with -Z macro-backtrace for more info) ``` --- When the primary span for a const error is the same as the first frame in the const error report, skip it. ``` error[E0080]: evaluation of constant value failed --> $DIR/issue-88434-removal-index-should-be-less.rs:3:24 | LL | const _CONST: &[u8] = &f(&[], |_| {}); | ^^^^^^^^^^^^^^ evaluation panicked: explicit panic | note: inside `f::<{closure@$DIR/issue-88434-removal-index-should-be-less.rs:3:31: 3:34}>` --> $DIR/issue-88434-removal-index-should-be-less.rs:10:5 | LL | panic!() | ^^^^^^^^ the failure occurred here = note: this error originates in the macro `$crate::panic::panic_2015` which comes from the expansion of the macro `panic` (in Nightly builds, run with -Z macro-backtrace for more info) ``` instead of ``` error[E0080]: evaluation of constant value failed --> $DIR/issue-88434-removal-index-should-be-less.rs:10:5 | LL | panic!() | ^^^^^^^^ explicit panic | note: inside `f::<{closure@$DIR/issue-88434-removal-index-should-be-less.rs:3:31: 3:34}>` --> $DIR/issue-88434-removal-index-should-be-less.rs:10:5 | LL | panic!() | ^^^^^^^^ note: inside `_CONST` --> $DIR/issue-88434-removal-index-should-be-less.rs:3:24 | LL | const _CONST: &[u8] = &f(&[], |_| {}); | ^^^^^^^^^^^^^^ = note: this error originates in the macro `$crate::panic::panic_2015` which comes from the expansion of the macro `panic` (in Nightly builds, run with -Z macro-backtrace for more info) ``` --- Revert order of constant evaluation errors Point at the code the user wrote first and std functions last. ``` error[E0080]: evaluation of constant value failed --> $DIR/const-errs-dont-conflict-103369.rs:5:25 | LL | impl ConstGenericTrait<{my_fn(1)}> for () {} | ^^^^^^^^ evaluation panicked: Some error occurred | note: called from `my_fn` --> $DIR/const-errs-dont-conflict-103369.rs:10:5 | LL | panic!("Some error occurred"); | ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ = note: this error originates in the macro `$crate::panic::panic_2015` which comes from the expansion of the macro `panic` (in Nightly builds, run with -Z macro-backtrace for more info) ``` instead of ``` error[E0080]: evaluation of constant value failed --> $DIR/const-errs-dont-conflict-103369.rs:10:5 | LL | panic!("Some error occurred"); | ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ Some error occurred | note: called from `<() as ConstGenericTrait<{my_fn(1)}>>::{constant#0}` --> $DIR/const-errs-dont-conflict-103369.rs:5:25 | LL | impl ConstGenericTrait<{my_fn(1)}> for () {} | ^^^^^^^^ = note: this error originates in the macro `$crate::panic::panic_2015` which comes from the expansion of the macro `panic` (in Nightly builds, run with -Z macro-backtrace for more info) ```
15 lines
629 B
Text
15 lines
629 B
Text
error[E0080]: evaluation of constant value failed
|
|
--> $DIR/const-unwrap.rs:6:18
|
|
|
|
|
LL | const BAR: i32 = Option::<i32>::None.unwrap();
|
|
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^ evaluation panicked: called `Option::unwrap()` on a `None` value
|
|
|
|
error[E0080]: evaluation of constant value failed
|
|
--> $DIR/const-unwrap.rs:10:18
|
|
|
|
|
LL | const BAZ: i32 = Option::<i32>::None.expect("absolutely not!");
|
|
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ evaluation panicked: absolutely not!
|
|
|
|
error: aborting due to 2 previous errors
|
|
|
|
For more information about this error, try `rustc --explain E0080`.
|