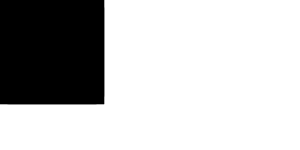
Remove `#[cfg(not(test))]` gates in `core` These gates are unnecessary now that unit tests for `core` are in a separate package, `coretests`, instead of in the same files as the source code. They previously prevented the two `core` versions from conflicting with each other.
423 lines
13 KiB
Rust
423 lines
13 KiB
Rust
//! # The Rust Core Library
|
|
//!
|
|
//! The Rust Core Library is the dependency-free[^free] foundation of [The
|
|
//! Rust Standard Library](../std/index.html). It is the portable glue
|
|
//! between the language and its libraries, defining the intrinsic and
|
|
//! primitive building blocks of all Rust code. It links to no
|
|
//! upstream libraries, no system libraries, and no libc.
|
|
//!
|
|
//! [^free]: Strictly speaking, there are some symbols which are needed but
|
|
//! they aren't always necessary.
|
|
//!
|
|
//! The core library is *minimal*: it isn't even aware of heap allocation,
|
|
//! nor does it provide concurrency or I/O. These things require
|
|
//! platform integration, and this library is platform-agnostic.
|
|
//!
|
|
//! # How to use the core library
|
|
//!
|
|
//! Please note that all of these details are currently not considered stable.
|
|
//!
|
|
// FIXME: Fill me in with more detail when the interface settles
|
|
//! This library is built on the assumption of a few existing symbols:
|
|
//!
|
|
//! * `memcpy`, `memmove`, `memset`, `memcmp`, `bcmp`, `strlen` - These are core memory routines
|
|
//! which are generated by Rust codegen backends. Additionally, this library can make explicit
|
|
//! calls to `strlen`. Their signatures are the same as found in C, but there are extra
|
|
//! assumptions about their semantics: For `memcpy`, `memmove`, `memset`, `memcmp`, and `bcmp`, if
|
|
//! the `n` parameter is 0, the function is assumed to not be UB, even if the pointers are NULL or
|
|
//! dangling. (Note that making extra assumptions about these functions is common among compilers:
|
|
//! [clang](https://reviews.llvm.org/D86993) and [GCC](https://gcc.gnu.org/onlinedocs/gcc/Standards.html#C-Language) do the same.)
|
|
//! These functions are often provided by the system libc, but can also be provided by the
|
|
//! [compiler-builtins crate](https://crates.io/crates/compiler_builtins).
|
|
//! Note that the library does not guarantee that it will always make these assumptions, so Rust
|
|
//! user code directly calling the C functions should follow the C specification! The advice for
|
|
//! Rust user code is to call the functions provided by this library instead (such as
|
|
//! `ptr::copy`).
|
|
//!
|
|
//! * Panic handler - This function takes one argument, a `&panic::PanicInfo`. It is up to consumers of this core
|
|
//! library to define this panic function; it is only required to never
|
|
//! return. You should mark your implementation using `#[panic_handler]`.
|
|
//!
|
|
//! * `rust_eh_personality` - is used by the failure mechanisms of the
|
|
//! compiler. This is often mapped to GCC's personality function, but crates
|
|
//! which do not trigger a panic can be assured that this function is never
|
|
//! called. The `lang` attribute is called `eh_personality`.
|
|
|
|
#![stable(feature = "core", since = "1.6.0")]
|
|
#![doc(
|
|
html_playground_url = "https://play.rust-lang.org/",
|
|
issue_tracker_base_url = "https://github.com/rust-lang/rust/issues/",
|
|
test(no_crate_inject, attr(deny(warnings))),
|
|
test(attr(allow(dead_code, deprecated, unused_variables, unused_mut)))
|
|
)]
|
|
#![doc(rust_logo)]
|
|
#![doc(cfg_hide(
|
|
no_fp_fmt_parse,
|
|
target_pointer_width = "16",
|
|
target_pointer_width = "32",
|
|
target_pointer_width = "64",
|
|
target_has_atomic = "8",
|
|
target_has_atomic = "16",
|
|
target_has_atomic = "32",
|
|
target_has_atomic = "64",
|
|
target_has_atomic = "ptr",
|
|
target_has_atomic_equal_alignment = "8",
|
|
target_has_atomic_equal_alignment = "16",
|
|
target_has_atomic_equal_alignment = "32",
|
|
target_has_atomic_equal_alignment = "64",
|
|
target_has_atomic_equal_alignment = "ptr",
|
|
target_has_atomic_load_store = "8",
|
|
target_has_atomic_load_store = "16",
|
|
target_has_atomic_load_store = "32",
|
|
target_has_atomic_load_store = "64",
|
|
target_has_atomic_load_store = "ptr",
|
|
))]
|
|
#![no_core]
|
|
#![rustc_coherence_is_core]
|
|
#![rustc_preserve_ub_checks]
|
|
//
|
|
// Lints:
|
|
#![deny(rust_2021_incompatible_or_patterns)]
|
|
#![deny(unsafe_op_in_unsafe_fn)]
|
|
#![deny(fuzzy_provenance_casts)]
|
|
#![warn(deprecated_in_future)]
|
|
#![warn(missing_debug_implementations)]
|
|
#![warn(missing_docs)]
|
|
#![allow(explicit_outlives_requirements)]
|
|
#![allow(incomplete_features)]
|
|
#![warn(multiple_supertrait_upcastable)]
|
|
#![allow(internal_features)]
|
|
#![deny(ffi_unwind_calls)]
|
|
#![warn(unreachable_pub)]
|
|
// Do not check link redundancy on bootstraping phase
|
|
#![allow(rustdoc::redundant_explicit_links)]
|
|
#![warn(rustdoc::unescaped_backticks)]
|
|
//
|
|
// Library features:
|
|
// tidy-alphabetical-start
|
|
#![feature(array_ptr_get)]
|
|
#![feature(asm_experimental_arch)]
|
|
#![feature(bigint_helper_methods)]
|
|
#![feature(bstr)]
|
|
#![feature(bstr_internals)]
|
|
#![feature(closure_track_caller)]
|
|
#![feature(const_carrying_mul_add)]
|
|
#![feature(const_eval_select)]
|
|
#![feature(core_intrinsics)]
|
|
#![feature(coverage_attribute)]
|
|
#![feature(disjoint_bitor)]
|
|
#![feature(internal_impls_macro)]
|
|
#![feature(ip)]
|
|
#![feature(is_ascii_octdigit)]
|
|
#![feature(lazy_get)]
|
|
#![feature(link_cfg)]
|
|
#![feature(non_null_from_ref)]
|
|
#![feature(offset_of_enum)]
|
|
#![feature(panic_internals)]
|
|
#![feature(ptr_alignment_type)]
|
|
#![feature(ptr_metadata)]
|
|
#![feature(set_ptr_value)]
|
|
#![feature(slice_as_array)]
|
|
#![feature(slice_as_chunks)]
|
|
#![feature(slice_ptr_get)]
|
|
#![feature(str_internals)]
|
|
#![feature(str_split_inclusive_remainder)]
|
|
#![feature(str_split_remainder)]
|
|
#![feature(ub_checks)]
|
|
#![feature(unchecked_neg)]
|
|
#![feature(unchecked_shifts)]
|
|
#![feature(utf16_extra)]
|
|
#![feature(variant_count)]
|
|
// tidy-alphabetical-end
|
|
//
|
|
// Language features:
|
|
// tidy-alphabetical-start
|
|
#![feature(abi_unadjusted)]
|
|
#![feature(adt_const_params)]
|
|
#![feature(allow_internal_unsafe)]
|
|
#![feature(allow_internal_unstable)]
|
|
#![feature(auto_traits)]
|
|
#![feature(cfg_sanitize)]
|
|
#![feature(cfg_target_has_atomic)]
|
|
#![feature(cfg_target_has_atomic_equal_alignment)]
|
|
#![feature(cfg_ub_checks)]
|
|
#![feature(const_precise_live_drops)]
|
|
#![feature(const_trait_impl)]
|
|
#![feature(decl_macro)]
|
|
#![feature(deprecated_suggestion)]
|
|
#![feature(doc_cfg)]
|
|
#![feature(doc_cfg_hide)]
|
|
#![feature(doc_notable_trait)]
|
|
#![feature(extern_types)]
|
|
#![feature(f128)]
|
|
#![feature(f16)]
|
|
#![feature(freeze_impls)]
|
|
#![feature(fundamental)]
|
|
#![feature(generic_arg_infer)]
|
|
#![feature(if_let_guard)]
|
|
#![feature(intra_doc_pointers)]
|
|
#![feature(intrinsics)]
|
|
#![feature(lang_items)]
|
|
#![feature(let_chains)]
|
|
#![feature(link_llvm_intrinsics)]
|
|
#![feature(macro_metavar_expr)]
|
|
#![feature(marker_trait_attr)]
|
|
#![feature(min_specialization)]
|
|
#![feature(multiple_supertrait_upcastable)]
|
|
#![feature(must_not_suspend)]
|
|
#![feature(negative_impls)]
|
|
#![feature(never_type)]
|
|
#![feature(no_core)]
|
|
#![feature(no_sanitize)]
|
|
#![feature(optimize_attribute)]
|
|
#![feature(prelude_import)]
|
|
#![feature(repr_simd)]
|
|
#![feature(rustc_allow_const_fn_unstable)]
|
|
#![feature(rustc_attrs)]
|
|
#![feature(rustdoc_internals)]
|
|
#![feature(simd_ffi)]
|
|
#![feature(staged_api)]
|
|
#![feature(stmt_expr_attributes)]
|
|
#![feature(strict_provenance_lints)]
|
|
#![feature(trait_alias)]
|
|
#![feature(transparent_unions)]
|
|
#![feature(try_blocks)]
|
|
#![feature(unboxed_closures)]
|
|
#![feature(unsized_fn_params)]
|
|
#![feature(with_negative_coherence)]
|
|
// tidy-alphabetical-end
|
|
//
|
|
// Target features:
|
|
// tidy-alphabetical-start
|
|
#![feature(aarch64_unstable_target_feature)]
|
|
#![feature(arm_target_feature)]
|
|
#![feature(avx512_target_feature)]
|
|
#![feature(hexagon_target_feature)]
|
|
#![feature(keylocker_x86)]
|
|
#![feature(loongarch_target_feature)]
|
|
#![feature(mips_target_feature)]
|
|
#![feature(powerpc_target_feature)]
|
|
#![feature(riscv_target_feature)]
|
|
#![feature(rtm_target_feature)]
|
|
#![feature(s390x_target_feature)]
|
|
#![feature(sha512_sm_x86)]
|
|
#![feature(sse4a_target_feature)]
|
|
#![feature(tbm_target_feature)]
|
|
#![feature(wasm_target_feature)]
|
|
#![feature(x86_amx_intrinsics)]
|
|
// tidy-alphabetical-end
|
|
|
|
// allow using `core::` in intra-doc links
|
|
#[allow(unused_extern_crates)]
|
|
extern crate self as core;
|
|
|
|
#[prelude_import]
|
|
#[allow(unused)]
|
|
use prelude::rust_2024::*;
|
|
|
|
#[macro_use]
|
|
mod macros;
|
|
|
|
#[unstable(feature = "assert_matches", issue = "82775")]
|
|
/// Unstable module containing the unstable `assert_matches` macro.
|
|
pub mod assert_matches {
|
|
#[unstable(feature = "assert_matches", issue = "82775")]
|
|
pub use crate::macros::{assert_matches, debug_assert_matches};
|
|
}
|
|
|
|
// We don't export this through #[macro_export] for now, to avoid breakage.
|
|
#[unstable(feature = "autodiff", issue = "124509")]
|
|
/// Unstable module containing the unstable `autodiff` macro.
|
|
pub mod autodiff {
|
|
#[unstable(feature = "autodiff", issue = "124509")]
|
|
pub use crate::macros::builtin::autodiff;
|
|
}
|
|
|
|
#[unstable(feature = "contracts", issue = "128044")]
|
|
pub mod contracts;
|
|
|
|
#[unstable(feature = "cfg_match", issue = "115585")]
|
|
pub use crate::macros::cfg_match;
|
|
|
|
#[macro_use]
|
|
mod internal_macros;
|
|
|
|
#[path = "num/shells/int_macros.rs"]
|
|
#[macro_use]
|
|
mod int_macros;
|
|
|
|
#[rustc_diagnostic_item = "i128_legacy_mod"]
|
|
#[path = "num/shells/i128.rs"]
|
|
pub mod i128;
|
|
#[rustc_diagnostic_item = "i16_legacy_mod"]
|
|
#[path = "num/shells/i16.rs"]
|
|
pub mod i16;
|
|
#[rustc_diagnostic_item = "i32_legacy_mod"]
|
|
#[path = "num/shells/i32.rs"]
|
|
pub mod i32;
|
|
#[rustc_diagnostic_item = "i64_legacy_mod"]
|
|
#[path = "num/shells/i64.rs"]
|
|
pub mod i64;
|
|
#[rustc_diagnostic_item = "i8_legacy_mod"]
|
|
#[path = "num/shells/i8.rs"]
|
|
pub mod i8;
|
|
#[rustc_diagnostic_item = "isize_legacy_mod"]
|
|
#[path = "num/shells/isize.rs"]
|
|
pub mod isize;
|
|
|
|
#[rustc_diagnostic_item = "u128_legacy_mod"]
|
|
#[path = "num/shells/u128.rs"]
|
|
pub mod u128;
|
|
#[rustc_diagnostic_item = "u16_legacy_mod"]
|
|
#[path = "num/shells/u16.rs"]
|
|
pub mod u16;
|
|
#[rustc_diagnostic_item = "u32_legacy_mod"]
|
|
#[path = "num/shells/u32.rs"]
|
|
pub mod u32;
|
|
#[rustc_diagnostic_item = "u64_legacy_mod"]
|
|
#[path = "num/shells/u64.rs"]
|
|
pub mod u64;
|
|
#[rustc_diagnostic_item = "u8_legacy_mod"]
|
|
#[path = "num/shells/u8.rs"]
|
|
pub mod u8;
|
|
#[rustc_diagnostic_item = "usize_legacy_mod"]
|
|
#[path = "num/shells/usize.rs"]
|
|
pub mod usize;
|
|
|
|
#[path = "num/f128.rs"]
|
|
pub mod f128;
|
|
#[path = "num/f16.rs"]
|
|
pub mod f16;
|
|
#[path = "num/f32.rs"]
|
|
pub mod f32;
|
|
#[path = "num/f64.rs"]
|
|
pub mod f64;
|
|
|
|
#[macro_use]
|
|
pub mod num;
|
|
|
|
/* The core prelude, not as all-encompassing as the std prelude */
|
|
|
|
pub mod prelude;
|
|
|
|
/* Core modules for ownership management */
|
|
|
|
pub mod hint;
|
|
pub mod intrinsics;
|
|
pub mod mem;
|
|
pub mod ptr;
|
|
#[unstable(feature = "ub_checks", issue = "none")]
|
|
pub mod ub_checks;
|
|
|
|
/* Core language traits */
|
|
|
|
pub mod borrow;
|
|
pub mod clone;
|
|
pub mod cmp;
|
|
pub mod convert;
|
|
pub mod default;
|
|
pub mod error;
|
|
pub mod marker;
|
|
pub mod ops;
|
|
|
|
/* Core types and methods on primitives */
|
|
|
|
pub mod any;
|
|
pub mod array;
|
|
pub mod ascii;
|
|
pub mod asserting;
|
|
#[unstable(feature = "async_iterator", issue = "79024")]
|
|
pub mod async_iter;
|
|
#[unstable(feature = "bstr", issue = "134915")]
|
|
pub mod bstr;
|
|
pub mod cell;
|
|
pub mod char;
|
|
pub mod ffi;
|
|
#[unstable(feature = "core_io_borrowed_buf", issue = "117693")]
|
|
pub mod io;
|
|
pub mod iter;
|
|
pub mod net;
|
|
pub mod option;
|
|
pub mod panic;
|
|
pub mod panicking;
|
|
#[unstable(feature = "pattern_type_macro", issue = "123646")]
|
|
pub mod pat;
|
|
pub mod pin;
|
|
#[unstable(feature = "random", issue = "130703")]
|
|
pub mod random;
|
|
#[unstable(feature = "new_range_api", issue = "125687")]
|
|
pub mod range;
|
|
pub mod result;
|
|
pub mod sync;
|
|
#[unstable(feature = "unsafe_binders", issue = "130516")]
|
|
pub mod unsafe_binder;
|
|
|
|
pub mod fmt;
|
|
pub mod hash;
|
|
pub mod slice;
|
|
pub mod str;
|
|
pub mod time;
|
|
|
|
pub mod unicode;
|
|
|
|
/* Async */
|
|
pub mod future;
|
|
pub mod task;
|
|
|
|
/* Heap memory allocator trait */
|
|
#[allow(missing_docs)]
|
|
pub mod alloc;
|
|
|
|
// note: does not need to be public
|
|
mod bool;
|
|
mod escape;
|
|
mod tuple;
|
|
mod unit;
|
|
|
|
#[stable(feature = "core_primitive", since = "1.43.0")]
|
|
pub mod primitive;
|
|
|
|
// Pull in the `core_arch` crate directly into core. The contents of
|
|
// `core_arch` are in a different repository: rust-lang/stdarch.
|
|
//
|
|
// `core_arch` depends on core, but the contents of this module are
|
|
// set up in such a way that directly pulling it here works such that the
|
|
// crate uses the this crate as its core.
|
|
#[path = "../../stdarch/crates/core_arch/src/mod.rs"]
|
|
#[allow(
|
|
missing_docs,
|
|
missing_debug_implementations,
|
|
dead_code,
|
|
unused_imports,
|
|
unsafe_op_in_unsafe_fn,
|
|
ambiguous_glob_reexports,
|
|
deprecated_in_future,
|
|
unreachable_pub
|
|
)]
|
|
#[allow(rustdoc::bare_urls)]
|
|
mod core_arch;
|
|
|
|
#[stable(feature = "simd_arch", since = "1.27.0")]
|
|
pub mod arch;
|
|
|
|
// Pull in the `core_simd` crate directly into core. The contents of
|
|
// `core_simd` are in a different repository: rust-lang/portable-simd.
|
|
//
|
|
// `core_simd` depends on core, but the contents of this module are
|
|
// set up in such a way that directly pulling it here works such that the
|
|
// crate uses this crate as its core.
|
|
#[path = "../../portable-simd/crates/core_simd/src/mod.rs"]
|
|
#[allow(missing_debug_implementations, dead_code, unsafe_op_in_unsafe_fn)]
|
|
#[allow(rustdoc::bare_urls)]
|
|
#[unstable(feature = "portable_simd", issue = "86656")]
|
|
mod core_simd;
|
|
|
|
#[unstable(feature = "portable_simd", issue = "86656")]
|
|
pub mod simd {
|
|
#![doc = include_str!("../../portable-simd/crates/core_simd/src/core_simd_docs.md")]
|
|
|
|
#[unstable(feature = "portable_simd", issue = "86656")]
|
|
pub use crate::core_simd::simd::*;
|
|
}
|
|
|
|
include!("primitive_docs.rs");
|