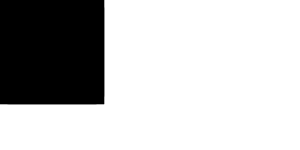
Remove unsafe `split_at_unchecked` and `split_at_mut_unchecked` in some slice `split_first_chunk`/`split_last_chunk` methods. Replace those calls with the safe `split_at` and `split_at_checked` where applicable. Add codegen tests to check for no panics when calculating the last chunk index using `checked_sub` and `split_at`
24 lines
867 B
Rust
24 lines
867 B
Rust
//@ compile-flags: -Copt-level=3
|
|
#![crate_type = "lib"]
|
|
|
|
// Check that no panic is generated in `split_at` when calculating the index for
|
|
// the tail chunk using `checked_sub`.
|
|
//
|
|
// Tests written for refactored implementations of:
|
|
// `<[T]>::{split_last_chunk, split_last_chunk_mut, last_chunk, last_chunk_mut}`
|
|
|
|
// CHECK-LABEL: @split_at_last_chunk
|
|
#[no_mangle]
|
|
pub fn split_at_last_chunk(s: &[u8], chunk_size: usize) -> Option<(&[u8], &[u8])> {
|
|
// CHECK-NOT: panic
|
|
let Some(index) = s.len().checked_sub(chunk_size) else { return None };
|
|
Some(s.split_at(index))
|
|
}
|
|
|
|
// CHECK-LABEL: @split_at_mut_last_chunk
|
|
#[no_mangle]
|
|
pub fn split_at_mut_last_chunk(s: &mut [u8], chunk_size: usize) -> Option<(&mut [u8], &mut [u8])> {
|
|
// CHECK-NOT: panic
|
|
let Some(index) = s.len().checked_sub(chunk_size) else { return None };
|
|
Some(s.split_at_mut(index))
|
|
}
|