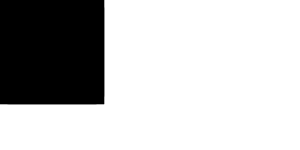
An assignment such as (a, b) = (b, c); desugars to the HIR { let (lhs, lhs) = (b, c); a = lhs; b = lhs; }; The repeated `lhs` leads to multiple Locals assigned to the same DILocalVariable. Rather than attempting to fix that, get rid of the debug info for these bindings that don't even exist in the program to begin with. Fixes #138198
18 lines
344 B
Rust
18 lines
344 B
Rust
//@ compile-flags: -g -Zmir-opt-level=0
|
|
|
|
#![crate_type = "lib"]
|
|
|
|
#[inline(never)]
|
|
fn swizzle(a: u32, b: u32, c: u32) -> (u32, (u32, u32)) {
|
|
(b, (c, a))
|
|
}
|
|
|
|
pub fn work() {
|
|
let mut a = 1;
|
|
let mut b = 2;
|
|
let mut c = 3;
|
|
(a, (b, c)) = swizzle(a, b, c);
|
|
println!("{a} {b} {c}");
|
|
}
|
|
|
|
// CHECK-NOT: !DILocalVariable(name: "lhs",
|